Data
In this lesson you will learn about the different types of data and how data is stored and organized within a computer program.
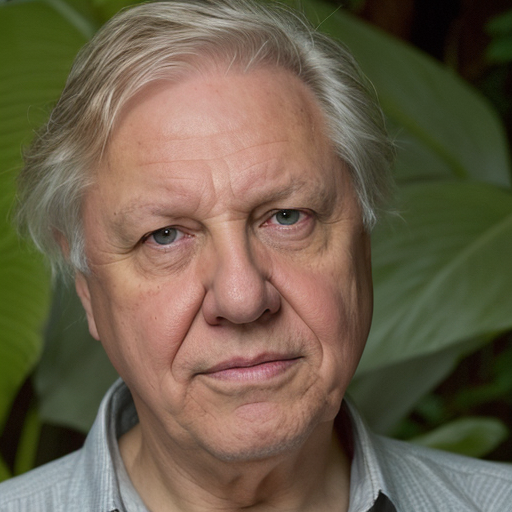
Historian
What is Data?
Data is information that is collected, stored, and analyzed to make decisions or understand things better. For example, if you've ever completed an online form, you probably have entered information such as your first and last name, your date of birth, whether you are married or not, your height, or other information about yourself. This data can then be used by companies to personalize your experience, improve services, or even make recommendations just for you.
There are four main types of data, known as data types.
- String - a string is text like
"Hello World"
. - Integer - an integer is a whole number like
203
. - Float - a float is a number with decimal point like
3.14
. - Boolean - a boolean is a value that can either be
True
orFalse
. Booleans are used for things like switches and checkboxes.
Notes About Strings
- Often you will see strings surrounded in quotes like (
"text"
or'text'
) to distinguish them from numbers or booleans. - You can use double quotes or single quotes for strings.
- When using quotes inside a string, it's best to use double quotes for the outer quotes and single quotes for the quotes inside the string. For example:
"He said, 'I think this is going to end well.'"
- In some cases you will have to use triple quotes if the string is longer than one line. See the example below.
- If you want to use double quotes in a string you can escape the quote by putting a backslash in front of it.
"He said, \"I think this is going to end well.\""
- A multi-line string should use triple quotes
""" Since this string is more than one line, we have to use triple quotes, otherwise it just may not work and you will get an error. """
- You can also use a \n to force a new line in a string
"Since this string is more than one line, \n we have to use triple quotes otherwise it just may \n not work and you will get an error."
Converting Data Types
You will often need to convert one data type to another. This is called typecasting.
str(45) # casting an integer to a string
int("456") # casting a string to an integer
int(45.0) # casting a float to an integer
float("44.0") # casting a float to a string
float(34) # casting a float to an integer
bool(1) # casting an integer to a boolean
bool(0) # casting an integer to a boolean
Storing Data in Variables
A variable is like a box that can store information. Variables are useful because they allow you store information for later use in the program. You can create a variable by typing its name followed by an equal sign and a data type. In user.py
, the variable named FirstName
is like a box that holds the string "Tutorial"
.
If you want to instruct the computer to show the contents of a variable on the screen, you can do so by using the print()
statement.
FirstName = "Tutorial"
LastName = "Doctor"
BirthYear = 1985
Height = 6.3
EmailAddress = "td@gmail.com"
IsAlive = True
print(FirstName)
print(EmailAddress)
DeviceName = "The g-phone"
OSVersion = 17.1
modelName = 'g-phone'
WiFi = True
AirplaneMode = False
Volume = 100
print(DeviceName)
print(OSVersion)
Take Action
Create a file named phone.py
in your code editor and copy the device.py
code above into the file. Change the variables in the file to represent the settings on your smart phone. Often the boolean data type for switches or checkboxes to turn things on or off. When creating each variable, think about what would be the best data type to use and why. What are the advantages or disadvantages?
Example Code
DeviceName = "iPhone XR"
OSVersion = '17.0.1'
ModelNumber= 'SASDU23A/B'
SerialNumber = '3223KK201XX'
WiFi = True
AirplaneMode = False
Volume = 100
Songs = 200
Videos = 100
Photos = 3000
Applications = 100
Capacity = '64GB'
Network = "Verizon"
AutomaticUpdates = False
Carplay = False
Brightness = 50.0
How to Name a Variable
Variable names should be descriptive. When naming a variable it should be clear what type information is being stored inside of the variable just by looking at the name. This helps you and other program easily read the program. The way you name a variable is called the naming convention.
The variables names below are NOT descriptive enough:
d = 365
n = "Joshua Hankins"
The variable names below ARE descriptive:
days_in_a_year = 365
my_brothers_name = "Joshua Hankins"
Using clear variable names makes your code easier to read and understand, both for yourself and for others who may read your code later.
Cases
With written language, letters can be either upper case or lower case. When making variable names in programming languages there are also several cases that can be used. Just as cases in English help you know when sentences begin, cases in a programming language help you know where new words begin in a variable name.
The Different Cases
Camel Case: The first letter of each word is capitalized except for the first letter of the name. camelCase
Pascal Case: The first letter of each word is capitalized, including for the first letter of the name. PascalCase
. Also called CapitalCamelCase
Snake Case: Each word is all lowercase and separated by underscores. snake_case
Constant Case: Each word is all uppercase and separated by underscores. CONSTANT_CASE
. Also called (MACRO_CASE or UPPER_CASE_SCREAM_CASE).
Here are some less common conventions:
Kebab Case: Uses a dash. kebab-case
. Also called (caterpillar-case, dash-case, hyphen-case, lisp-case, spinal-case or css-case)
Train Case: Each word is all uppercase and separated by a dash. TRAIN-CASE
. Also called COBOL-CASE
Flat Case: Each word is all lowercase and there is no separation between words. flatcase
. Also called mumblecase
THINK...
- What is the disadvantage of using single letters for variable names?
- When might one case be preferred over another?
Arithmetic
You can use a programming language to perform mathematical calculations like addition, subtraction, multiplication and division. Here are some of the basic arithmetic operations you can perform on integers and floats:
- Addition (
+
) - adds two numbers together - Subtraction (
-
) - subtracts one number from another - Multiplication (
*
) - multiplies one number by another - Division (
/
) divides one number by another
The following examples use arithmetic operators to operate on numbers. In birthday_calculator.py
you can calculate your age by subtracting your birth year from the current year. In gpa_calculator.py
you can calculate your GPA by dividing the sum of your grades by the number of grades you have.
birthYear = 1985
currentYear = 2024
age = currentYear - birthYear
print(age)
total_grades = 90 + 80 + 93 + 95
gpa = total_grades / 4
print(gpa)
Note
In gpa_calculator.py
the abbreviation of gpa
is fine for a variable name because that abbreviation is known to mean grade point average.
Take Action
Think about things in your life that require math. For example, calculating a bill, converting between units (like meters to feet), or finding the average of a set of numbers.
Create a file named your_thing_calculator.py
and write a program that stores the data in variables, does some calculation, and prints the results.
Example Code
water = 50.05
electric = 100.00
phone = 200.00
car_note = 400.00
months_in_a_year = 12
total_bills_a_year = (water + electric + phone + car_note) * months_in_a_year
print(total_bills_a_year)
Joining Strings
You can also use the +
operator to join strings together. This is called concatenation. Concatenation can be used for things like joining a first, middle and last name together or to join a street number, city, state and zip of an address into one string. In concatenation.py
you can see that FirstName
and LastName
are concatenated and stored in a variable named FullName
. This would make the value of FullName
equal to "TutorialDoctor"
.
FirstName = "Tutorial"
LastName = "Doctor"
FullName = FirstName + LastName
print(FullName)
To add a space between the names, you can concatenate the strings with another string that has a single space.
FullName = FirstName + " " + LastName
print(FullName)
Note that if you want to concatenate a string and a integer together, you have to cast one to the data type of the other.
age = 34
name = "Tutorial Doctor"
print(name + " is " + str(age) + " years old")
String Interpolation
You can inject data of another type into a string using interpolation. Sometimes you may be given data in a numerical format and you need to put it into a string, like the street number for an address.
One way to inject data into a string in Python is by using f-strings, which is a string with the letter f
before it. In interpolation.py
the FirstName
, LastName
, Age
and Height
variables are injected into in a variable named Bio
. When you print Bio
you get "Your name is Tutorial Doctor and your age is 38 and your height is 5.2"
You can also use %s
formatting and .format()
to do string interpolation in Python.
FirstName = "Tutorial"
LastName = "Doctor"
Age = 38
Height = 5.2
Bio = f"Your name is {FirstName} {LastName} and your age is {Age} and your height is {Height}"
print(Bio)
StreetNumber = 123
StreetName = "Cool Street"
ApartmentNumber = 33
State = "California"
StateAbbreviation = "CA"
ZIP = 50210
Country = "USA"
Address = f"{StreetNumber} {StreetName} Apartment #{ApartmentNumber} {State}, {StateAbbreviation} {ZIP} {Country}"
print(Address)
FirstName = "Tutorial"
LastName = "Doctor"
FullName = "%s %s" %(FirstName,LastName,)
print(FullName)
FirstName = "Tutorial"
LastName = "Doctor"
FullName = "{} {}".format(FirstName,LastName)
print(FullName)
What is a Data Structure?
A data structure is a specific way of organizing data in a program. The two main data structures in programming are the list
and the dictionary
.
List
If you've ever used a grocery list or a todo list, you are familiar with how useful lists are when organizing things. Lists are also very useful in programs to organize data.
A list lets you store multiple items in a specific order and refer to them by their position. Each item in the list has an index which its position in the list. In grocery_list.py
, a list of strings is created and stored in a well-named variable called groceryList
. The index of the string milk
is 0
and you can access the milk using the its index. You can also add new items to a list, update items in a list, remove a specific item from a list or remove the last item a the list.
groceryList = ['milk','eggs','cheese','bread']
print(groceryList[0]) # Grabbing the first item from the list
groceryList.append('pizza')# Adding a new item to the list
groceryList[2] = 'water' # Squeezing in an item into the list at position 2
groceryList.remove('bread') # Removing an item from the list by its name
groceryList.pop() # Removing the last item you added from the list
# A list of tasks I need to do
todoList = [
"fold the clothes",
"repair the garage door",
"go on a date",
"wash the car",
"take out the trash"
]
# A list of 7-digit phone numbers
phoneNumbers = [
1234567890,4346786565,6585657434,3447776567
]
Dictionary
Another useful data structure is the dictionary. A dictionary is like a list where each item in the dictionary has a key and a value. In fruit_dictionaries.py
the key color
has the value red
. You can access an item in a dictionary by using the key of the item. A dictionary is also called an Object, Key-value Pair, Hash Map, Hash Table, Hash Set or Associative Array. It is called an associative array because each item in the dictionary (the key) is "associated" with some other data (the value).
# A dictionary with data about an apple
apple = {
"color": "red",
"shape": "round",
"weight": .33,
"texture": "smooth"
}
# A dictionary with data about an orange
orange = {
"color": "orange",
"shape": "round",
"weight": .44,
"texture": "bumpy"
}
print(apple['color']) # get an item from the dictionary
print(orange['color'])
apple['name'] = 'granny smith' # add an item to the dictionary
apple['weight'] = .35 # change an item in the dictionary
del apple['name'] # remove an item from the dictionary
# A dictionary of words and their associated definitions
wordDictionary = {
"a": "the first letter of the english alphabet",
"aa": "basaltic lava having a rough, broken surface",
"aaa": """a small battery that is used in small electronic devices and
that is standardized at 44 mm in length and 10.5 mm in diameter"""
}
# Three dictionaries that contain information about users of an application
user = {
"id": 1,
"email": "myemail@gmail.com",
"password:": "123456password",
"role": "admin"
}
user2 = {
"id": 2
"email": "theiremail@gmail.com",
"password:": "password123456",
"role": "staff"
}
user3 = {
"id": 3
"email": "regularemail@gmail.com",
"password:": "123password456",
"role": "basic"
}
Notes about Lists and Dictionaries
- You may have noticed that each item in the list or dictionary was written on a new line. This isn't required but it is done to make the program more readable.
- Note that you can put lists inside of dictionaries or dictionaries inside of lists, or you can put lists inside of lists or dictionaries inside of dictionaries!
- A list is created using square brackets
[]
and a dictionary is created using braces{}
. - It is common to see a list of dictionaries used in a program.
Combining Lists and Dictionaries
In many computer programs you will see lists and dictionaries used together to represent data for a real-world object. In users_list.py
you will see a "list of users" that could represent people that use a social media platform.
users = [
{
"id": 1,
"email": "hank@gmail.com",
"password:": "98765password",
"role": "clerk"
},
{
"id": 2,
"email": "sam@gmail.com",
"password:": "54321password",
"role": "admin"
},
{
"id": 3,
"email": "rachel@gmail.com",
"password:": "1234567password",
"role": "manager"
},
]
groceryList = [
{'name': 'milk', 'quantity': 2, 'brand': 'Fairlife'},
{'name': 'eggs', 'quantity': 24, 'brand': 'Vital Farms'},
{'name': 'cheese', 'quantity': 2, 'brand': 'Kraft'},
{'name': 'bread', 'quantity': 2, 'brand': 'Ezekiel 4:9'}
]
myInfo = {
"name": "Tutorial Doctor",
"age": 39,
"height": 6.2,
"hobbies": ['drawing','origami','coding'],
"attributes": {"eyeColor": "brown", "hairColor": "black"}
}
Take Action
- Try adding, removing and updating items in the dictionaries above
Summary
Congratulations! You now know what data is, the 4 types of data, and how to convert them from one to the other. You've learned how to store data in variables and how to organize data into lists and dictionaries. In the next lesson you will learn how give the computer instructions on what to do with your data.
Terms
- data - bits of information
- data type - the type of data
- variable - container that stores data
- concatenation - joining two strings
- interpolation - injecting data into a string
- list - A way structure data in sequential order
- dictionary - A way to structure key-value data
Your Turn!
- Open your code editor
- Create a file called
user_data.py
- Create a variable
user_data
with an empty dictionary - Store the following data in the variable
first_name
,last_name
,middle_name
,birthday
,gender
,other names
,previous names
,emails
,phone numbers
,relationships
,education experiences
,work experiences
,blood info
,websites
,about info
,favorite quote
- Run the program and see if there are any errors
THINK...
What are the appropriate data-types to use for the data?
What type of apps would a variable like this be used for?
Example Code
user_data = {
"profile_data": {
"name": {
"full_name": "Tutorial Doctor Smith",
"first_name": "Tutorial Doctor",
"middle_name": "",
"last_name": "Smith"
},
"emails": {
"emails": [
"ramonsuthers@gmail.com",
"josiahrapha09@yahoo.com"
],
"previous_emails": [
"Tutorial Doctor.j.smith@facebook.com"
],
"pending_emails": [
],
"ad_account_emails": [
]
},
"birthday": {
"year": 1986,
"month": 4,
"day": 9
},
"gender": {
"gender_option": "MALE"
},
"previous_names": [
],
"other_names": [
],
"relationship": {
"status": "Married",
"partner": "Sarah Jones",
"anniversary": {
"year": 2011,
"month": 3,
"day": 20
}
},
"education_experiences": [
],
"work_experiences": [
{
"employer": "TD LLC",
"title": "Full Stack Web Developer",
"location": "Richmond, CA",
"description": "I built apps."
}
],
"interested_in": [
"FEMALE"
],
"blood_info": {
"blood_donor_status": "unregistered"
},
"websites": [
{
"address": "https://www.churchofthelatterrain.com/"
}
],
"phone_numbers": [
{
"phone_type": "Mobile",
"phone_number": "+14044059442",
"verified": True
}
],
"about_me": "One day a high school history teacher of mine made a statement that would change my life's direction..."
}
}
Discovery
The dictionary you created is based on data that represents a user on Facebook