Libraries
In this lesson you will learn how libraries can be used to save you time.
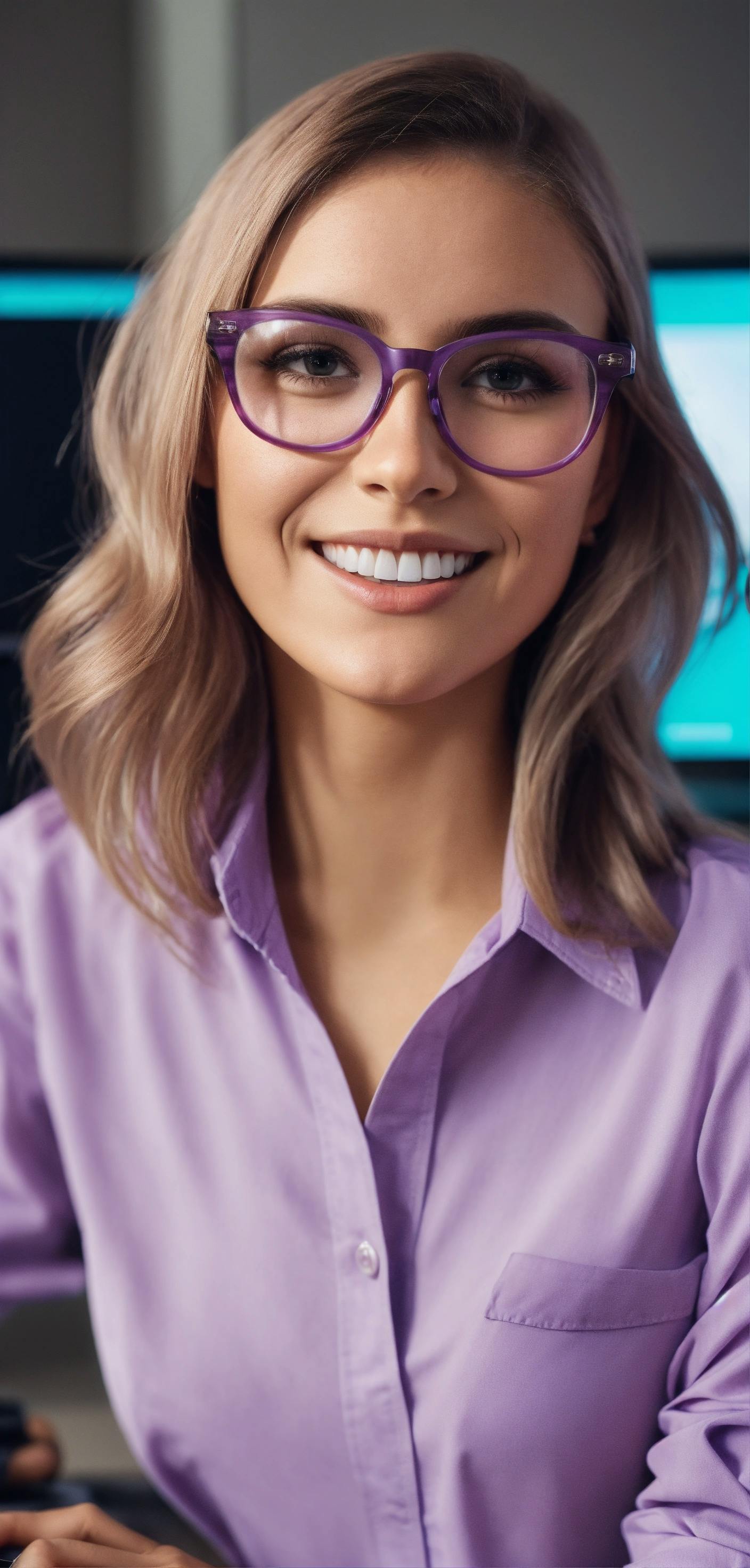
Junior Developer
What is a Library?
Alright, imagine you're building a cool treehouse. You could cut down your own trees, saw the wood, hammer every nail, and design the whole thing from scratch. But, wouldn’t it be easier if someone handed you a kit with all the wood pre-cut, the nails organized, and even some design templates to help you along? That’s kinda what a library in programming is. It’s a collection of pre-written code that you can use to solve common problems, so you don’t have to start from zero every time.
When to Use a Library?
Think of a library like a toolkit for your coding projects. Whenever you’re working on something and realize, "Hmm, someone else has probably done this before," that's when you should reach for a library. Need to handle dates and times? There's a library for that. Want to make web requests? Yep, there is a library for that too. Libraries save you time and energy by providing you with tried-and-true solutions for common coding challenges.
Built-in Python Libraries
Python comes with its own toolbox of built-in libraries. These are like the essentials you need for everyday tasks. Here are a few:
math
: Need to do some complex math? This library has functions for trigonometry, logarithms, factorials, etc.datetime
: This one helps you work with dates and times. Perfect for when you need to figure out how many days until your next vacation!os
: Want to interact with your computer’s operating system?os
lets you work with file paths, directories, and more.
Since these libraries are built into Python, you don’t need to install anything. Just import them and you’re good to go!
Third-Party Libraries
Third-party libraries are libraries made by other people (thank you, other people!). Python’s community is super active, so there’s a library for almost anything you can think of.
requests
: Need to make HTTP requests?requests
is your best friend for interacting with web APIs.Pillow
: Working with images?Pillow
lets you manipulate them, from resizing to adding filters.NumPy
: This one’s a must for data crunching, helping you handle large arrays and matrices of numbers with ease.
Package Managers
So, how do you get these third-party libraries? Enter the package manager. Think of it as a personal shopper for your coding needs. It fetches libraries (packages) from the internet, installs them, and even helps keep them updated.
Popular Package Managers
For Python, the go-to package manager is pip
. It’s super simple to use:
pip install requests
: This command downloads and installs therequests
library for you.
If you’re working with more complex projects, you might run into pipenv
or poetry
, which help manage not just libraries but also virtual environments and dependencies (basically making sure all your tools work well together).
Making Your Own Python Package
Feeling ambitious? You can make your own Python package and share it with the world! Here’s the basic idea:
- Organize Your Code: Put your code into a proper directory structure.
- Create a
setup.py
File: This file contains information about your package, like its name, version, and dependencies. - Distribute It: Once your package is ready, you can upload it to PyPI (Python Package Index), which is like the app store for Python libraries.
And just like that, you’re contributing to the coding community, helping others just like those library creators helped you!
Examples
import math
print(math.pi)
print(math.sqrt(49))
print(math.abs(-29))
import urllib.request
import ssl
url = "https://jsonplaceholder.typicode.com/todos/"
context = ssl._create_unverified_context()
response = urllib.request.urlopen(url,context=context)
print(response.getcode())
print(response.read().decode())
import re
sentence = "Sam's personal email address is sam@gmail.com. His work email is sam@busfactories.org. His mobile phone number is 404-494-4949 and his home phone is 344-343-3434. He lives at 42344 Haring Way Rd."
phone_numbers = re.findall("\d{3}-\d{3}-\d{3}",sentence)
email_addresses = re.findall("[a-zA-Z0-9]+@[a-zA-Z0-9]+\.[a-zA-Z]{3}",sentence)
print(phone_numbers)
print(email_addresses)
import os
for root, dirs, files in os.walk("."):
path = root.split(os.sep)
print((len(path) - 1) * '---', os.path.basename(root))
for file in files:
print(len(path) * '---', file)
from datetime import date
today = date.today()
print("Current year:", today.year)
print("Current month:", today.month)
print("Current day:", today.day)
import shutil
shutil.copy('./file.txt','/home')
Third Party Examples
from faker import Faker
fake = Faker()
print("Name: \n" + fake.name())
print("Address: \n" + fake.address())
print("Text: \n" + fake.text())
import tkinter as tk
window = tk.Tk()
window.title('Counting Seconds')
button = tk.Button(window, text='Stop', width=25, command=window.destroy)
button.pack()
window.mainloop()
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots() # Create a figure containing a single Axes.
ax.plot([1, 2, 3, 4], [1, 4, 2, 3]) # Plot some data on the Axes.
plt.show()
Popular Package Managers
Every programming language has its own package manager
Your Turn!
Let's build a app!
- Open your code editor
- Step 2
- Step 3
THINK...
Something to think about
Solution
Discovery
Something you should have gained from this exercise