Loops
In this lesson you will learn how to repeat instructions
What is a loop?
A loop lets you repeat instructions over and over. There are two main types of loops in programming, the for loop and the while loop.
For Loop
A for loop lets you repeat instructions until you check everything off. You can use it to keep doing something until a counter hits a certain number, you reach the last item in a backpack (like a list!), or you finish going through a whole dictionary.
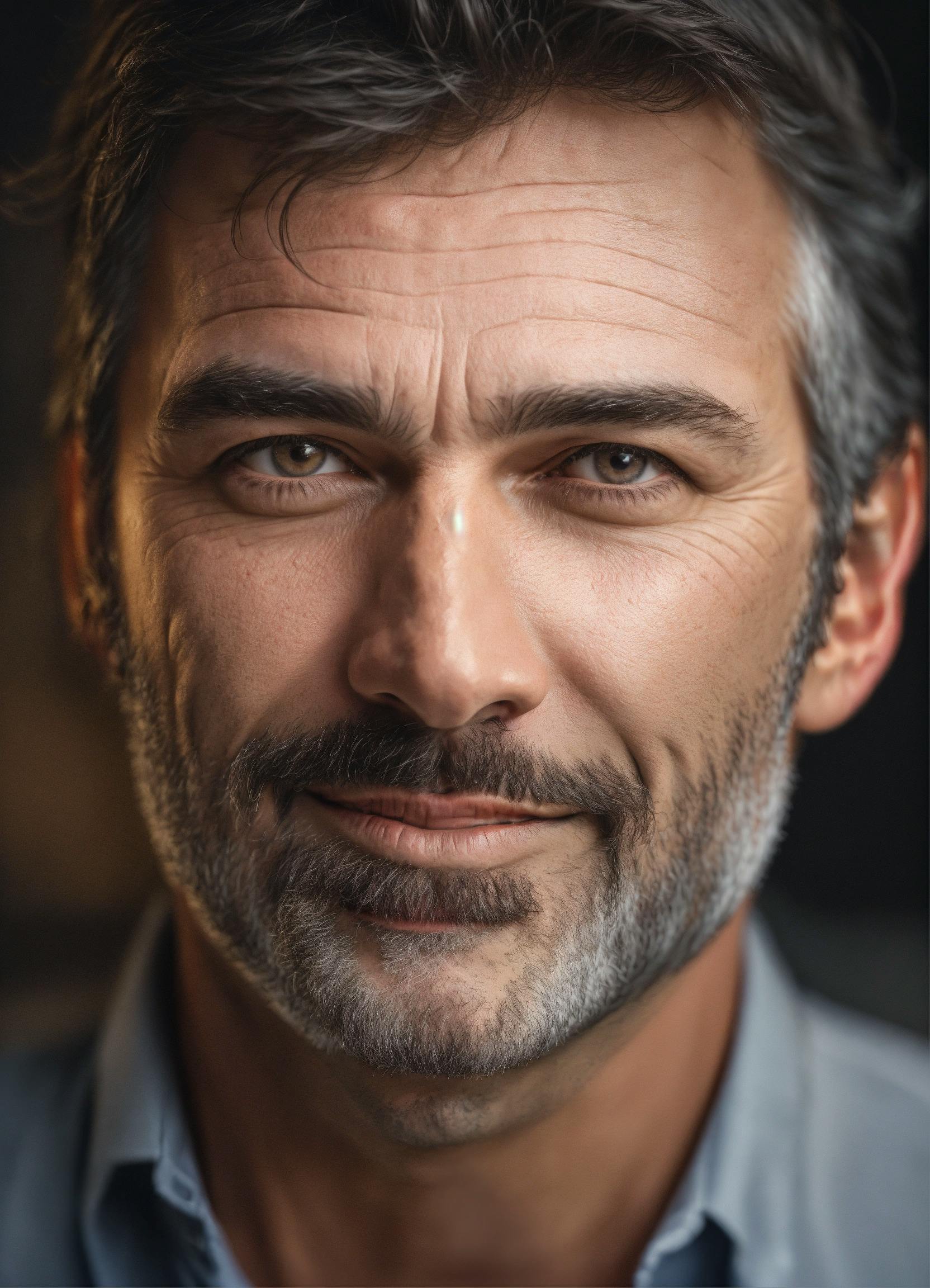
Junior Developer
The range()
function creates a collection of numbers in a given range, for example, between 1 and 10. In count.py
below, we tell the computer to grab numbers from 0 all the way to 59 (but not 60!), and print each one out as it goes. In names_list_loop.py
, the loop goes through a list of names, one by one, until it reaches the end. In words_dict_loop.py
we do something similar with a dictionary.
for i in range(0,60):
print(i)
t = 0
for i in range(0,100):
t+=1
print(t)
names = ['robert','susan','joe','cynthia']
for name in names:
print(name)
words = {
'a': 'first letter',
'b': 'second letter',
'c': 'third letter'
}
for k,v in enumerate(words.values()):
print(k,v)
While Loop
Unlike for loop, a while loop keeps doing something as long as a certain condition is true.
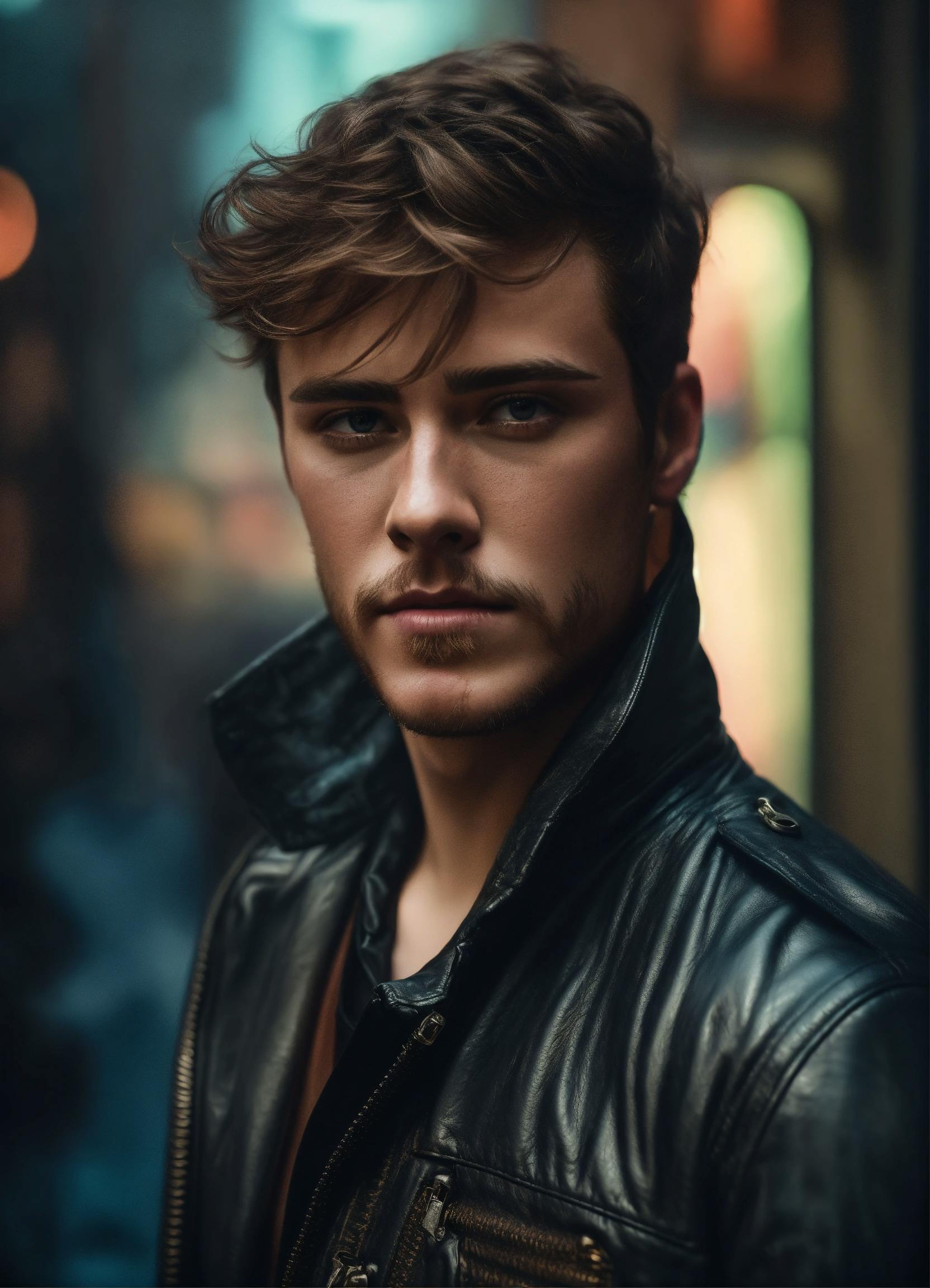
Junior Developer
Imagine you need a timer in your program. In timer.py
, we create a variable named timer
and set its value to 0
. This is where the timer starts. Then, as long as the timer is less than 300, the loop keeps printing the timer value. Once it hits 300 or more, the loop stops.
timer = 0
while timer < 300:
timer = timer + 1
print(timer)
In the code above, timer
will be printed as long as it is less than 300
, once it is greater than or equal to 300
, it will stop printing.
To make sure the loop stops at some point, we need to make sure the loop condition changes eventually. Otherwise, it'll keep repeating forever and the program will get stuck! That's why in most while loops, you'll see something that changes the condition (like increasing the timer in this example). This way, the loop knows when to quit!
Loop through a list
You can also loop through a list using a for loop. The loop will repeat until you get to the end of the list.
# Create a list of strings
names = ["Joe","Same","Bog"]
# For every string in the list, print the string
for name in names:
print(name)
Another way to loop through a list is to use the range function.
names = ["Joe","Same","Bog"]
for i in range(0,len(names)):
print(names[i])
The len()
function gets the length of a list.
Loop through a dictionary
You can loop through dictionary as well. To loop over a dictionary while getting the key and value, you must use the enumerate function of Python.
# Create a dictionary
data = {'color': 'red'}
# Using i as an iterator, for every key and
# value in the dictionary, print the key and value
for i, (key,value) in enumerate(data.items()):
print(key,value)
Notice the difference when you do it this way:
# Create a dictionary
data = {'color': 'red'}
# Using i as an iterator, for every key and
# value in the dictionary, print the key and value
for (key,value) in enumerate(data.items()):
print(key,value)
NOTE
Notice that we add one to the timer on every iteration of the loop, if we didn't eventually make the while loop greater than 300, it would keep repeating, because timer would always be less than 300.
Your Turn!
Let's build a Chatbot!
- Open your code editor
- Step 2
- Step 3
THINK...
Something to think about
Solution
memory = ['null']
username = input('What is your name? ')
# Fuzzy Data
cold = (0,60)
high = (10,60)
it = 32 # current value
import sys
ai_age = 1
ai_creationDay = 'Wednesday September 02, 2015'
ai_name = 'TD001' #__ means DON'T' TOUCH!
# But you can touch it anyway :)
info = {
'name': ai_name,
'age': ai_age,
'created': ai_creationDay
}
ai_weight = str(sys.getsizeof(info)) + ' bytes'
ai_info = f"""My name is {ai_name}. I am {ai_age}. I was created on
{ai_creationDay} by The Tutorial Doctor. I am currently {ai_weight})"""
def Main():
displayCommands()
running=True
while running:
command=input('Type a command %s: '%username).upper()
if command=='STOP':
print(f'{ai_name} Terminated')
return False
prompt(command)
def prompt(c):
greet(c)
checkMemory(c)
dates(c)
eraseConsole(c)
askInfo(c)
randomStatement(c)
train(c)
Fuzz(c)
import random,datetime,os
def displayCommands():
print('\n')
print('Console: erase,stop')
print('Memory: mem,memory,int()')
print('Personality: rant')
print('Info: info,age,name,birthday,weight')
print('Date: date,day,month,year')
print('Ask: good/evil,')
print('FuzzyLogic: cold?,high?')
print('\n')
def greet(c):
greetings=['HELLO','HI','HOLA','KONNICHIWA']
if c in greetings:
greeting = greetings[random.randint(0,3)].capitalize() + ' ' + username
block = (c,greeting)
print(greeting)
memory.append(block)
def checkMemory(c):
if c in ['MEMORY','MEM']:
print(str(len(memory)) + ' ' + str(memory))
try:
print(memory[int(c)])
except:
None
def askInfo(c):
# perhaps use all() or any()?
# not working (and)
if c == 'AGE':
print(f'I am {ai_age}.')
if c == 'NAME':
print(f'My name is {ai_name}')
if c in ['BIRTH','BIRTHDAY']:
print('I was created on {ai_creationDay}')
if ai_creationDay == datetime.date.today().strftime('%A %B %d, %Y'):
print("It's my creationday!")
if c in ['SIZE','WEIGHT','SZ']:
print('I am ' + ai_weight)
if c in ['INFO','NFO']:
print(ai_info)
if c in ['COMMANDS']:
displayCommands()
#subtract add dates?
def dates(c):
"""
#%b month abbreviation
#%B full month name
#%h month abbreviation
#%y two digit year
#%Y four digit year
#%a day of the week abbreviation
#%A day of the week
#%d date
#%m minutes
#%s seconds
#%s am/pm
#%c date & time
"""
today = datetime.date.today()
year = today.year
month = today.month
day = today.day
# Add times later
if c=='DAY':
print('It is ' + today.strftime('%A ') + username)
elif c=='MONTH':
print('It is ' + today.strftime('%B ') + username)
elif c=='YEAR':
print('It is ' + today.strftime('%Y ') + username)
elif c=='TIME':
pass
# print datetime.time()
elif c == 'TODAY':
pass
elif c == 'DATE':
print('It is ' + today.strftime('%A %B %d, %Y ') + username)
def eraseConsole(c):
if c in ['...','ER','ERASE']:
print('o')
clear = lambda: os.system('clear')
clear()
def randomStatement(c):
if c in ['RAN','RANT','RANDOM']:
s1 = 'Follow me on Twitter @TutorialDoctor'
s2 = 'Python is a cool programming language!'
s3 = 'What goes up, must come down. Unless it has 0 gravity of course.'
s4 = 'The grass is always greener on the other side. So true for deserts.'
s5 = 'Haha, that is such a blooper.'
s6 = f'WHO YOU CALLING FAT!. I am only {ai_weight}'
s = [s1,s2,s3,s4,s5,s6]
print(random.choice(s))
def train(c):
moral_data = {'GOOD':True,'EVIL':False}
if c in moral_data:
print(moral_data[c])
block = (c,moral_data[c])
memory.append(block)
# Fuzzy Logic
def Membership(x,List):
"Returns the membership of a value in a list."
top=(float(x)-List[0])
bottom=(List[-1]-List[0])
M= top/bottom
return M
def Is(x,List):
"Returns true if a value is in the value range of a list"
#If a value is greater than the first item in a list..
if x >= List[0]:
#And if it is smaller than the last item in the list...
if x<= List[-1]:
#print the membership of the item in the list...
print(Membership(x,List))
#And return True
return True
# No else statement is needed since the return statement will exit the function.
#Print the membership and return False if the above condition is false.
print(Membership(x,List))
return False
def Fuzz(c):
if c == 'COLD?':
print(Is(it,cold))
if c == 'HIGH?':
print(Is(it,high))
Main()
Discovery
Something you should have gained from this exercise