Course Summary
Let's sum up what programming is all about.
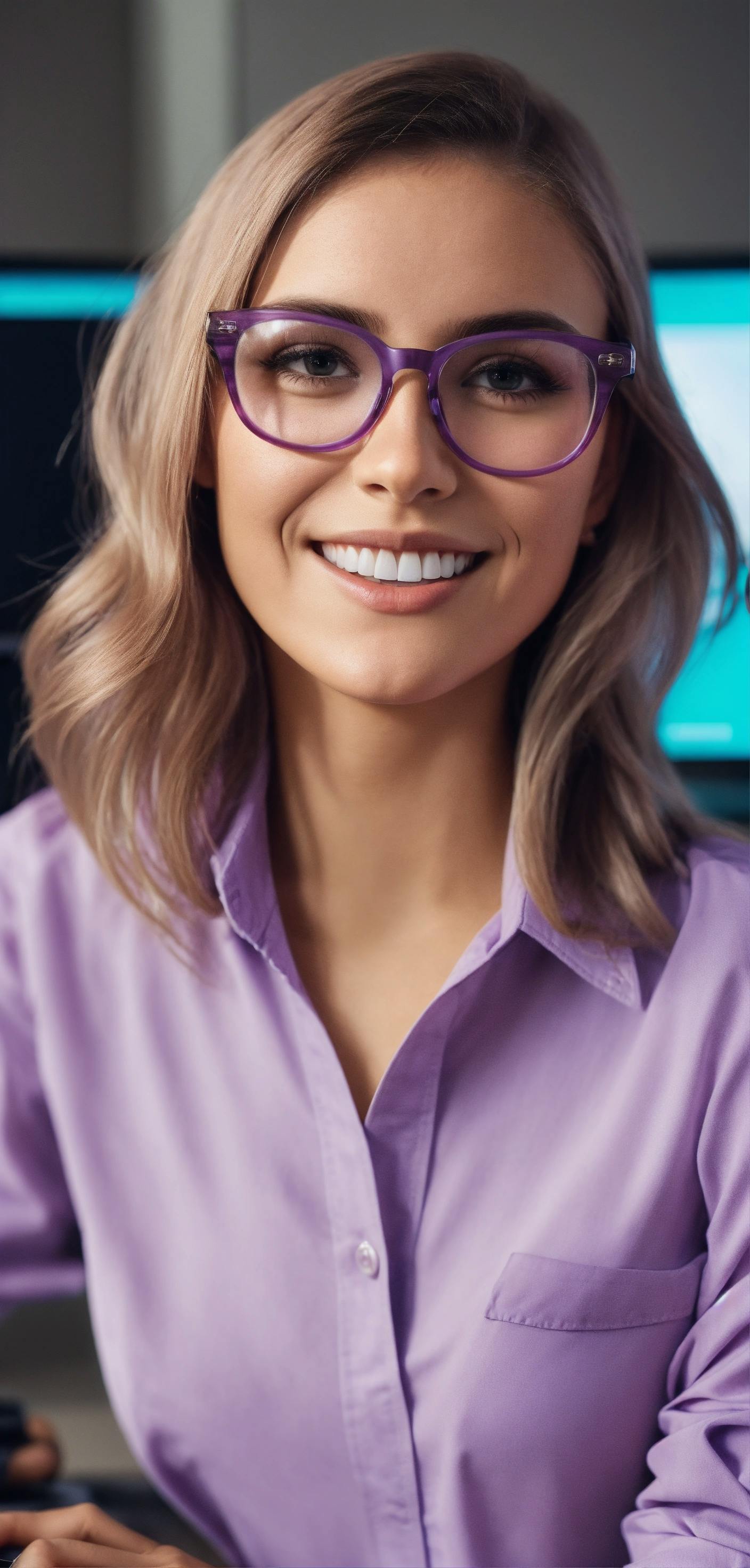
Jessica
Junior Developer
Junior Developer
Programming helps you do tasks faster by giving computer instructions. Information is saved in variables, which can be manipulated with statements, which can be repeated with loops which can be grouped into functions and classes and used to turn business rules into business logic.
This is how it all works:
- First, create a file and open it with a code editor.
- Then, create variables to store data.
- Type statements to manipulate the data and repeat statements using loops.
- Group related statements into functions.
- Group related functions and the data they manipulate into classes.
- Import libraries to save you time.
- Use everything above to translate business rules into code.
- Create a user interface to interact with the code, like clicking a button to trigger a function.
- Permanently store your data in a database so you don’t lose it.
- Use algorithms to do things more efficiently.
- Make the app secure so no one can steal the data.
- Find an app you like and try to re-create the user interface, data and business logic of the app.
- Test to make sure everything works.
- Repeat this entire process for another app to gain experience.
View the code
python
def sum(a,b):
return a + b
def difference(a,b):
return a - b
def product(a,b):
return a * b
def quotient(a,b):
if b!= 0:
return a / b
else:
return "Can't divide by zero"
def remainder(a,b):
if a % b == 0:
return 'even'
else:
return 'odd'
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n-1)
import unittest
class TestMath(unittest.TestCase):
def test_addition(self):
result = sum(2,3)
self.assertEquals(result, 5)
def test_subtraction(self):
result = difference(5,3)
self.assertEquals(result, 2)
def test_multiplication(self):
result = product(2,3)
self.assertEquals(result, 6)
def test_division(self):
result = quotient(26,2)
self.assertEquals(result, 13)
def test_remainder(self):
result = remainder(12,2)
self.assertEquals(result, 'even')
def test_factorial(self):
result = factorial(5)
self.assertEquals(result, 120)
class MathWork():
# You can move the functions to this class
pass
if __name__ == '__main__':
unittest.main()