Business Rules
Now that you have the core concepts down, you can combine them to model the rules of a business and actually get things done!
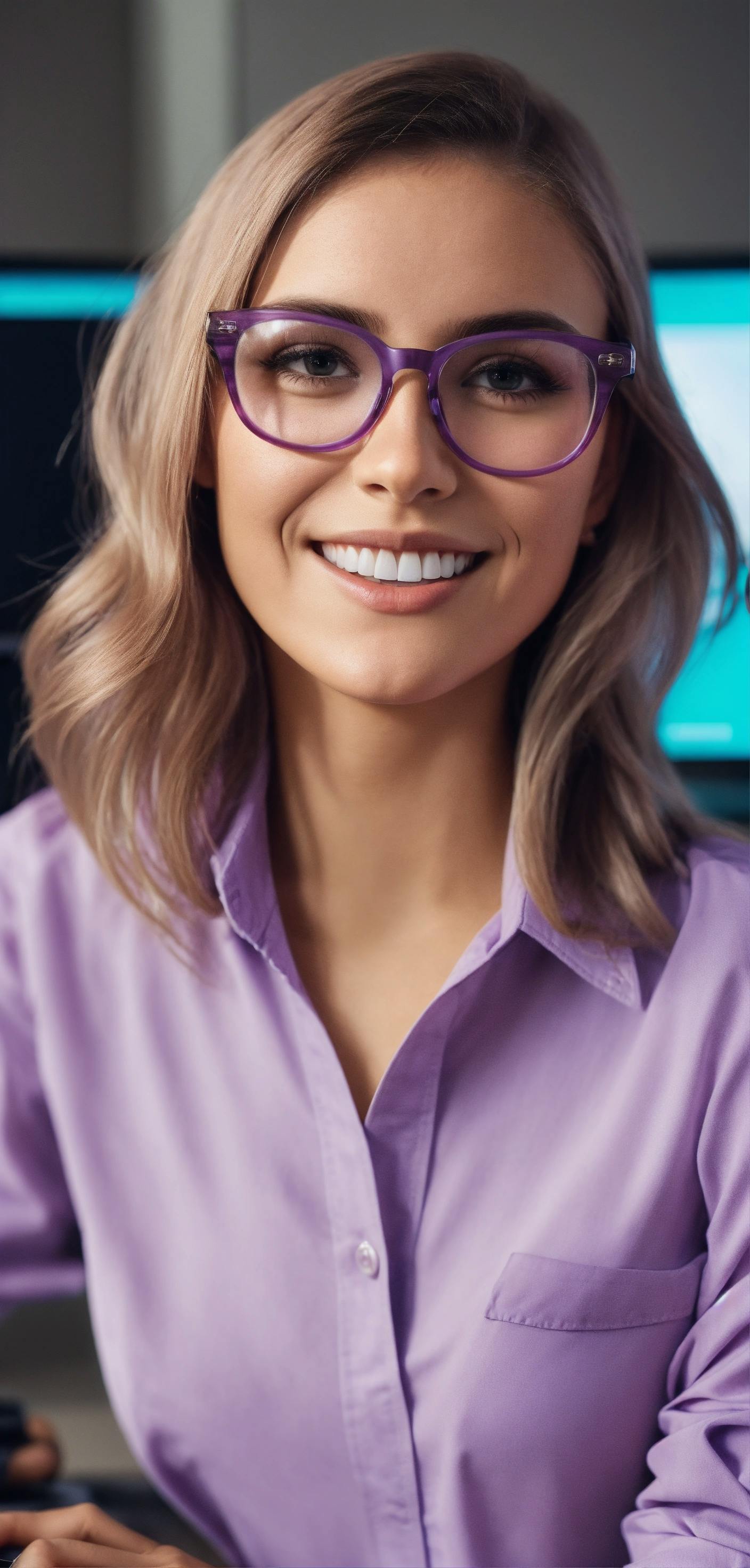
Junior Developer
What Are Businesses Rules and Business Logic?
Business rules are the rules that govern a business. These rules are regulations, policies, and other information about how the business does business. Think of them as a company's playbook. A company might have rules that say "customers have to be 18 to rent a car" or "employees get two weeks of vacation a year." These rules are what make one company different from another. Netflix and Disney+ are both streaming services, but they have different rules about what shows they offer, how much they charge, and so on.
Business logic is created when you turn those rules into computer code. It's the part of the program that figures out how to follow the playbook. If you're building a website for a car rental company, the business logic would be the code that checks a customer's age, figures out the rental price, and makes sure the car is available. It's like teaching a computer to understand and follow the company's rules.
So, business rules are the ideas, and business logic is how you make those ideas happen in code.
Constraint rules
These rules specify the limitations or constraints that must be adhered to in order to ensure data integrity and consistency. For example, a constraint rule might specify that a customer's age must be between 18 and 65.
class Customer:
def __init__(self):
self.age = 20
self.credit_score = 500
def passesAgeCheck(self):
if not self.age in range(18,65):
return False
else:
return True
customer = Customer()
print(customer.passesAgeCheck())
Decision rules
These rules specify how decisions should be made within a business process. For example, a decision rule might specify that if a customer's credit score is below a certain threshold, they should be denied a loan.
class Customer:
def __init__(self):
self.age = 20
self.credit_score = 500
def passesAgeCheck(self):
if not self.age in range(18,65):
return False
else:
return True
def checkCreditScore(self):
if self.credit_score < 700:
self.approved_for_loan = False
print("This customer is NOT approved for a loan")
else:
self.approved_for_loan = True
print("This customer is approved for a loan")
return self.approved_for_loan
customer = Customer()
print(customer.passesAgeCheck())
customer.checkCreditScore()
Calculation rules
These rules specify how calculations should be performed within a business process. For example, a calculation rule might specify that a discount should be applied to a customer's order if they spend more than $100.
class Customer():
def __init__(self,name):
self.age = 20
self.credit_score = 500
self.name = name
self.orders = []
class OrderDB():
db = []
@classmethod
def create(cls,order):
cls.db.append(order)
class Order():
def __init__(self,customer=None,total=0):
self.customer = customer
self.total = total
def create(self):
order = {"customer": self.customer, "total": self.total}
if order['total'] > 100:
order['discount'] = .10
self.customer.orders.append(order)
OrderDB.create(order)
def __repr__(self):
return str({"customer": self.customer.name})
customer_1 = Customer("Glen")
order_1 = Order(customer_1)
order_1.create()
print(order_1)
print(OrderDB.db)
Validation rules
These rules specify the conditions that must be met in order for a piece of data or transaction to be considered valid. For example, a validation rule might specify that an email address must be in a valid format in order to be considered valid.
import re
class Customer():
def __init__(self,name,email):
self.age = 20
self.credit_score = 500
self.name = name
self.email = email
self.orders = []
def validemail(self):
regex = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,7}\b'
if(re.fullmatch(regex, self.email)):
print("Valid Email")
return True
else:
print("Invalid Email")
return False
class OrderDB():
db = []
@classmethod
def create(cls,order):
cls.db.append(order)
class Order():
def __init__(self,customer=None,total=0):
self.customer = customer
self.total = total
def create(self):
order = {"customer": self.customer, "total": self.total}
if order['total'] > 100:
order['discount'] = .10
self.customer.orders.append(order)
OrderDB.create(order)
def __repr__(self):
return str({"customer": self.customer.name})
customer_1 = Customer("Glen",'glen@gmail.com')
order_1 = Order(customer_1)
order_1.create()
print(order_1)
print(OrderDB.db)
print(customer_1.validemail())
Authorization rules
These rules specify the actions that a user is authorized to perform. For example, an authorization rule might specify that a customer service representative is authorized to provide refunds but not to approve credit applications.
Inferencing rules
These rules are used to infer new information from existing data. For example, a rule might infer that if a customer has bought a product from a particular category, they might be interested in similar products from that category.
Data governance rules
These rules help to ensure that data is accurate, consistent, and secure. For example, a data governance rule might specify that all sensitive data must be encrypted when it is stored.
How to Turn Rules into Logic
- Think in terms of data and functions.
- What data is involved in the rule and what data structures would be used to store the data? Look for any nouns in the rule.
- What functions or actions are taken? Look for any verbs in the rule.
- If you notice that there are several related functions, consider adding them to a class.
- Consider the conditions in which something does or doesn't happen
- Are there any collections of data? You will use loops for actions that need to repeat or when iterating through collections of data
Other things to consider
- Consider groups of classes that may end up needing to be a library
- Consider what libraries (built-in or third-party) that may solve a problem before you do.
- If a class could very well be it's own app, consider making it a microservice.
Your Turn!
Let's build an e-commerce app!
- Open your code editor
- Step 2
- Step 3
THINK...
Something to think about
Solution
# customer's age must be between 18 and 65.
# If a customer's credit score is below a certain threshold, they should be denied a loan.
# a discount should be applied to a customer's order if they spend more than $100.
import re
class Customer:
def __init__(self,name):
self.age = 20
self.name = name
self.email = ""
self.credit_score = 500
self.approved_for_loan = False
self.role = "manager"
self.orders = []
self.policy = EditPolicy
def passesAgeCheck(self):
if not self.age in range(18,65):
return False
else:
return True
def checkCreditScore(self):
if self.credit_score < 700:
self.approved_for_loan = False
print("This customer is NOT approved for a loan")
else:
self.approved_for_loan = True
print("This customer is approved for a loan")
return self.approved_for_loan
def validEmail(self):
email_validate_pattern = r"^\S+@\S+\.\S+$"
match = re.match(email_validate_pattern, self.email)
if match is not None:
return match
else:
return "This your email is invalid"
def advancedValidEmail(self):
email_validate_pattern = r"^[a-z0-9!#$%&'*+/=?^_`{|}~-]+(?:\.[a-z0-9!#$%&'*+/=?^_`{|}~-]+)*@(?:[a-z0-9](?:[a-z0-9-]*[a-z0-9])?\.)+[a-z0-9](?:[a-z0-9-]*[a-z0-9])?$"
match = re.match(email_validate_pattern, self.email)
if match is not None:
return match
else:
return "This your email is invalid"
def __repr__(self):
return str({"name": self.name, "creditScore": self.credit_score,"approvedForLoan": self.approved_for_loan,"orders": self.orders})
class OrderDB():
orders = []
@classmethod
def create(cls,data):
cls.orders.append(data)
class Order():
def __init__(self,customer=None,total=0):
self.customer = customer
self.total = total
def create(self):
order = {"customer": self.customer, "total": self.total}
if order['total'] > 100:
order['discount'] = .10
self.customer.orders.append(order)
OrderDB.create(order)
def __repr__(self):
return str({"customer": self.customer.name})
class Policy():
name = "BasePolicy"
class EditPolicy(Policy):
name = "EditPolicy"
canReFund = True
canApprove = False
print(EditPolicy.name)
customer = Customer("Joe")
customer.email ="joe@gmail.com"
print(customer.passesAgeCheck())
print(customer.checkCreditScore())
order = Order(customer,300)
order.create()
print(OrderDB.orders)
print(customer.validEmail())
customer.email ="joegmail.com"
print(customer.advancedValidEmail())
print(customer.policy.name)